Часто использую в javascript
Работа со строками
Поиск позиции вхождения подстроки в строке
strng.indexOf(substr)
Расщепить строку на элементы
domain.split('.')[0]
Работа с типами данных
Проверить переменная массив — да-нет?
supposed instanceof Array
Проверить есть ли в переменной что-нибудь или она типа object
(typeof selectedCity === 'undefined' || typeof selectedCity === 'object')
Работа с массивами
Задать переменную-массив
var arr = [1, -1, 2, -2, 3];
Проверить наличие элемента в массиве
supposed.indexOf(this.getArea()) //если нет - возвратит -1
Отфильтровать массив
var positiveArr = arr.filter(function(number) {
return number > 0;
});
Если надо прервать перебор элементов в forEach, то нужно использовать оператор some
res._embedded.items.some(function (item) { if(!item.is_completed) { $('#card_holder').before(modal_okno); return; } })
Работа с датами
var dt = new Date();
Получить дату в формате unix: new Date().getTime()
console.log(dt.getDay()+’.’+dt.getMonth()+’.’+dt.getFullYear()+’ ‘+dt.getHours()+’:’+dt.getMinutes());
Преобразовать дату в строку
(new Date()).toDateString()
Сравнить две даты
<pre class="EnlighterJSRAW" data-enlighter-language="generic" data-enlighter-theme=" data-enlighter-highlight=" data-enlighter-linenumbers=" data-enlighter-lineoffset=" data-enlighter-title=" data-enlighter-group=">dateRefresh();
/*$(document).on('blur','.hasDatepicker',function(){
dateRefresh();
});*/
$(document).on('change','.hasDatepicker',function(){
dateRefresh();
});
function dateRefresh() {
var datetime_regex = /(dd)/(dd)/(dddd)/;
var usinput = datetime_regex.exec($('.hasDatepicker').val());
if(usinput !== null){
var first_datetime = new Date(usinput[3], usinput[1]-1, usinput[2], '23', '59');
var second_datetime = new Date();
if(first_datetime.getTime() < second_datetime.getTime()) {
var newdate = new Date(second_datetime.getFullYear(),second_datetime.getMonth(),second_datetime.getDate()+1,second_datetime.getHours(),second_datetime.getMinutes());
var befdig = '';
var newmonth = newdate.getMonth()+1;
if(newdate.getMonth()<10) befdig = '0';
console.log($('.hasDatepicker').val());
$('.hasDatepicker').val(befdig+newmonth+'/'+newdate.getDate()+'/'+newdate.getFullYear());
}
}
}</pre>
dateRefresh(); /*$(document).on('blur','.hasDatepicker',function(){ dateRefresh(); });*/ $(document).on('change','.hasDatepicker',function(){ dateRefresh(); }); function dateRefresh() { var datetime_regex = /(dd)/(dd)/(dddd)/; var usinput = datetime_regex.exec($('.hasDatepicker').val()); if(usinput !== null){ var first_datetime = new Date(usinput[3], usinput[1]-1, usinput[2], '23', '59'); var second_datetime = new Date(); if(first_datetime.getTime() < second_datetime.getTime()) { var newdate = new Date(second_datetime.getFullYear(),second_datetime.getMonth(),second_datetime.getDate()+1,second_datetime.getHours(),second_datetime.getMinutes()); var befdig = ''; var newmonth = newdate.getMonth()+1; if(newdate.getMonth()<10) befdig = '0'; console.log($('.hasDatepicker').val()); $('.hasDatepicker').val(befdig+newmonth+'/'+newdate.getDate()+'/'+newdate.getFullYear()); } } }
Работа с url
Определить хвост url за доменом
window.location.pathname window.open('url') - открыть в отдельной вкладке
Перезагрузить страницу
window.location.reload();
Определение/объявление/задание своих функций
Записать в переменную экземпляр функции
var selector = new AddressSelector();
Работа с JSON
JSON.parse(settings.user_logins)[current_user];
Диалоги
Вывести диалог подтверждения
confirm('Вы уверены что хотите изменить поле?')
Специальные символы
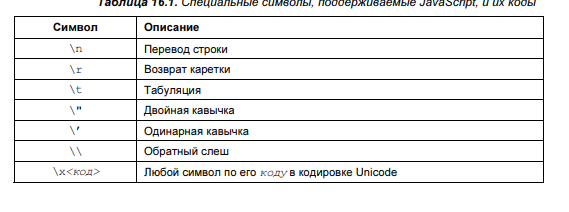
Обработка исключений javascript
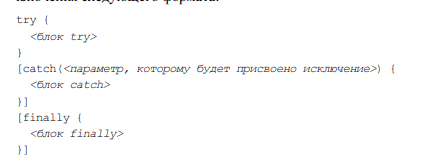
Пример
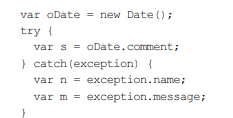
Работа с переменными и инициализацией
window.Filter = new createFilter(); $(function () { initListeners(); Filter.init(); });
Какие исключения поддерживает js
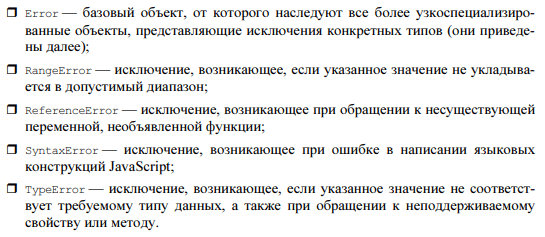
Обработка событий в js
Можно посмотреть в книге Laravel.быстрая разработка со страницы[1] 261
Управление элементами страницы и доступ к ним
со страницы [1] 239
Пример хорошо структурированного скрипта
здесь реализован лендинг в Opencart с помощью javascript класса filter (самописного)
/******/ (function(modules) { // webpackBootstrap /******/ // The module cache /******/ var installedModules = {}; /******/ /******/ // The require function /******/ function __webpack_require__(moduleId) { /******/ /******/ // Check if module is in cache /******/ if(installedModules[moduleId]) { /******/ return installedModules[moduleId].exports; /******/ } /******/ // Create a new module (and put it into the cache) /******/ var module = installedModules[moduleId] = { /******/ i: moduleId, /******/ l: false, /******/ exports: {} /******/ }; /******/ /******/ // Execute the module function /******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__); /******/ /******/ // Flag the module as loaded /******/ module.l = true; /******/ /******/ // Return the exports of the module /******/ return module.exports; /******/ } /******/ /******/ /******/ // expose the modules object (__webpack_modules__) /******/ __webpack_require__.m = modules; /******/ /******/ // expose the module cache /******/ __webpack_require__.c = installedModules; /******/ /******/ // define getter function for harmony exports /******/ __webpack_require__.d = function(exports, name, getter) { /******/ if(!__webpack_require__.o(exports, name)) { /******/ Object.defineProperty(exports, name, { /******/ configurable: false, /******/ enumerable: true, /******/ get: getter /******/ }); /******/ } /******/ }; /******/ /******/ // getDefaultExport function for compatibility with non-harmony modules /******/ __webpack_require__.n = function(module) { /******/ var getter = module && module.__esModule ? /******/ function getDefault() { return module['default']; } : /******/ function getModuleExports() { return module; }; /******/ __webpack_require__.d(getter, 'a', getter); /******/ return getter; /******/ }; /******/ /******/ // Object.prototype.hasOwnProperty.call /******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); }; /******/ /******/ // __webpack_public_path__ /******/ __webpack_require__.p = "; /******/ /******/ // Load entry module and return exports /******/ return __webpack_require__(__webpack_require__.s = 176); /******/ }) /************************************************************************/ /******/ ({ /***/ 176: /***/ (function(module, exports, __webpack_require__) { __webpack_require__(5); /***/ }), /***/ 5: /***/ (function(module, exports) { (function($) { var extendParams = window.filterExtendParams || {}; var createFilter = function () { this.fetching = false; this.filters = {}; this.ocfilter = {}; this.order = null; this.sort = null; this.page = 1; this.limit = 15; }; var filterWrap = '.filter-items-list'; createFilter.prototype.setFilterData = function (data) { this.filters = data; return this; }; createFilter.prototype.setOcFilterData = function (data) { this.ocfilter = data; return this; }; createFilter.prototype.setSortOrder = function (sort, order) { this.order = order; this.sort = sort; return this; }; createFilter.prototype.setPage = function (data) { this.page = parseInt(data); return this; }; createFilter.prototype.setLimit = function (data) { this.limit = parseInt(data); return this; }; createFilter.prototype.getParams = function () { const filters = {}; for(var filter in this.filters) { if (this.filters[filter]) filters[filter] = this.filters[filter]; } return { filters: filters, filter_ocfilter: this.ocfilter.filter_ocfilter, filter_selected: this.ocfilter.selected, path: this.ocfilter.path, order: this.order, sort: this.sort, page: this.page, limit: this.limit }; }; createFilter.prototype.fetch = function (params, callback) { var self = this; if (this.fetching) return; this.fetching = true; params = params || {}; params.replaceList = params.hasOwnProperty('replaceList') ? params.replaceList : true; var controller = window.controller || 'product/category'; if (!params.keepPage) { this.setPage(1); } var data = this.getParams(); data.output = 'json'; data.route = controller; $.extend(data, extendParams); this.productListAction('hide'); $.getJSON('/index.php', data , function (json) { self.fetching = false; history.pushState(null, '', json.url); var $content = $(json.content); var $findContent = $content.is(filterWrap) ? $content : $content.find(filterWrap); if (params.replaceList) { $(filterWrap).html($findContent.html()); } else { $(filterWrap).append($findContent.html()); } $('.content .pagination').html(json.pagination); if(json.pl_top){ $('#product_collection .pl_top').html(json.pl_top); } if(json.pl_bottom){ $('#product_collection .pl_bottom').html(json.pl_bottom); } if(json.breadcrumbs){ $('.breadcrumbs').html(json.breadcrumbs); } self.productListAction('show'); if (callback !== void 0 && typeof callback === 'function') { callback(json); } }); }; createFilter.prototype.productListAction = function (action) { if (action === 'show') { $('#product_collection .filter-loading').remove(); } else { $('#product_collection').append( $('<div />') .addClass('filter-loading') .html('<img src="catalog/view/theme/aztek/image/loading.gif" height="25" />') ); } return this; }; createFilter.prototype.init = function () { if (Utils.$_GET('page')) { Filter.setPage(parseInt(Utils.$_GET('page'))); } if (Utils.$_GET('limit')) { Filter.setLimit(parseInt(Utils.$_GET('limit'))); } else if ($('#add_more').length) { Filter.setLimit(parseInt($('#add_more').attr('data-limit'))); } if (Utils.$_GET('sort')) { Filter.setSortOrder(Utils.$_GET('sort'), Utils.$_GET('order')); } else { var $selected = $('#sort-order .active-result'); Filter.setSortOrder($selected.attr('data-sort'), $selected.attr('data-order')); } }; var initListeners = function() { $(document).on('click', '#sort-order a', function (e) { e.preventDefault(); var $this = $(this); if ($this.hasClass('active-result')) { var order = ($this.attr('data-order') === 'ASC') ? 'DESC' : 'ASC'; $this.attr('data-order', order); return Filter.setSortOrder($this.attr('data-sort'), order).fetch(); } $this.addClass('active-result').siblings().removeClass('active-result'); Filter.setSortOrder($this.attr('data-sort'), $this.attr('data-order')).fetch(); }); $(document).on('click', '.pagination .pager a', function (e) { e.preventDefault(); return Filter.setPage($(this).attr('data-page')).fetch({ keepPage: true }); }); $(document).on('click', '#add_more', function (e) { e.preventDefault(); Filter.setPage($(this).attr('data-page')); if ($(this).attr('data-limit')) { Filter.setLimit($(this).attr('data-limit')); } Filter.fetch({ replaceList: false, keepPage: true }); }); }; window.Filter = new createFilter(); $(function () { initListeners(); Filter.init(); }); })(jQuery); /***/ }) /******/ });
Откуда брал материал
[1] Ларавел. быстрая разработка.